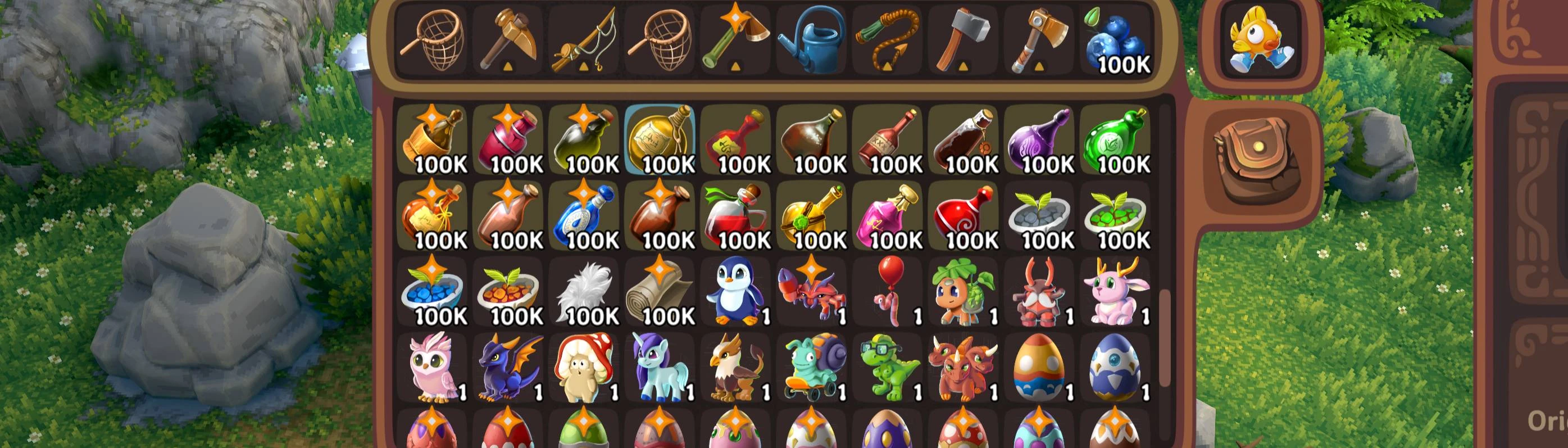
Easy x4 dmg Cheat Mode DLL without BepInEx
-
Endorsements
-
Unique DLs--
-
Total DLs--
-
Total views--
-
Version1.1
Documentation
Readme
View as plain text
using System;
using System.Collections;
using System.Collections.Generic;
using System.Runtime.CompilerServices;
using TMPro;
using UnityEngine;
using UnityEngine.InputSystem;
using UnityEngine.UI;
// Token: 0x020008F7 RID: 2295
public partial class ConsoleUI : UIGameObjectWindow, IGameUIListener, IGameUIGetter
{
// Token: 0x060032DA RID: 13018 RVA: 0x000E8214 File Offset: 0x000E6414
protected void Update()
{
if (Keyboard.current == null && Gamepad.current == null)
{
return;
}
// Check for F12 or Gamepad activation
if ((Keyboard.current != null && Keyboard.current.f12Key.wasPressedThisFrame) ||
(Gamepad.current != null && Gamepad.current.leftStickButton.wasPressedThisFrame && Gamepad.current.rightStickButton.wasPressedThisFrame))
{
if (this.m_panel.activeSelf)
{
base.Close(); // Close the console UI
}
else
{
this.Open(); // Open the console UI
this.PlayerGameUI.Player.GetComponent<ControlContextManager>().SetConsoleActive(true);
this.m_input.FixedSelect(); // Focus the input field
}
}
// Close console with Escape or Gamepad Button East
if (((Keyboard.current != null && Keyboard.current.escapeKey.wasPressedThisFrame) ||
(Gamepad.current != null && Gamepad.current.buttonEast.wasPressedThisFrame)) && this.m_panel.activeSelf)
{
base.Close();
}
// Navigate suggestions with Tab or Gamepad D-Pad Up
if (this.IsOpen &&
((Keyboard.current != null && Keyboard.current.tabKey.wasPressedThisFrame) ||
(Gamepad.current != null && Gamepad.current.dpad.up.wasPressedThisFrame)) &&
this.m_suggestedWords.Count > 0)
{
if (this.m_selectedSuggestion != -1)
{
this.m_suggestionItems[this.m_selectedSuggestion].GetComponent<Image>().color = Color.white;
}
this.m_selectedSuggestion = (this.m_selectedSuggestion + 1) % this.m_suggestedWords.Count;
this.m_suggestionItems[this.m_selectedSuggestion].GetComponent<Image>().color = Color.blue;
}
// Apply suggestion with Shift or Gamepad D-Pad Right
if (this.IsOpen &&
((Keyboard.current != null && Keyboard.current.leftShiftKey.wasPressedThisFrame) ||
(Gamepad.current != null && Gamepad.current.dpad.right.wasPressedThisFrame)) &&
this.m_selectedSuggestion != -1)
{
this.m_suggestionItems[this.m_selectedSuggestion].GetComponent<Image>().color = Color.white;
this.ApplyLatestSuggestion();
}
// Command history navigation with Up Arrow or Gamepad D-Pad Down
if (this.m_input.isFocused)
{
if (((Keyboard.current != null && Keyboard.current.upArrowKey.wasPressedThisFrame) ||
(Gamepad.current != null && Gamepad.current.dpad.down.wasPressedThisFrame)) &&
this.m_commandHistory.Count > 0)
{
this.m_historyIndex--;
if (this.m_historyIndex < 0)
{
this.m_historyIndex = this.m_commandHistory.Count - 1;
}
this.m_input.text = this.m_commandHistory[this.m_historyIndex];
}
// Clear input with Gamepad D-Pad Left
if (Gamepad.current != null && Gamepad.current.dpad.left.wasPressedThisFrame)
{
this.m_input.text = string.Empty;
}
// Remove backquote character if typed
if (Keyboard.current != null && Keyboard.current.backquoteKey.wasPressedThisFrame)
{
this.m_input.text = this.m_input.text.Replace("`", "");
}
}
// Execute command with Enter or Gamepad Button South
if (this.IsOpen &&
((Keyboard.current != null && Keyboard.current.enterKey.wasPressedThisFrame) ||
(Gamepad.current != null && Gamepad.current.buttonSouth.wasPressedThisFrame)))
{
if (this.m_selectedSuggestion != -1)
{
this.m_suggestionItems[this.m_selectedSuggestion].GetComponent<Image>().color = Color.white;
this.ApplyLatestSuggestion();
}
this.DoCommand();
}
}
}
```
//--- Modified `DamageUtil` Class ---
//```csharp
using System;
using System.Linq;
using Unity.Mathematics;
using Unity.VisualScripting;
using UnityEngine;
// Token: 0x02000A09 RID: 2569
[Inspectable]
public class DamageUtil
{
public static float CalcDamageToWorldObject(PlayerController player, int2 targetTile, InventoryItem tool)
{
if (tool == null)
{
return 0f;
}
TileData data = player.Level.GetData(targetTile);
HealthData healthData = (data != null) ? data.GetCustomData<HealthData>() : null;
if (healthData == null)
{
return 0f;
}
TileData data2 = player.Level.GetData(targetTile);
if (data2 == null)
{
return 0f;
}
float result;
if (data2.Type.Classification.Contains("Mineable"))
{
result = DamageUtil.GetMineableDamage(data2.Type, healthData, tool);
}
else if (data2.Type.Classification.Contains("Tree"))
{
result = DamageUtil.GetTreeDamage(data2.Type, healthData, player, targetTile, tool);
}
else
{
result = ToolUpgradeConstants.GetUpgradedToolDamage(tool, data2.Type) * 5f;
}
return result;
}
private static float GetMineableDamage(WorldItemsData worldItemTarget, HealthData health, InventoryItem tool)
{
float num = ToolUpgradeConstants.GetUpgradedToolDamage(tool, worldItemTarget);
return num * 5f;
}
private static float GetTreeDamage(WorldItemsData worldItemTarget, HealthData health, PlayerController player, int2 targetTile, InventoryItem tool)
{
float num = ToolUpgradeConstants.GetUpgradedToolDamage(tool, worldItemTarget);
num *= DamageUtil.GetTreeAgeMultiplier(player.Level, targetTile);
return num * 5f;
}
private static float GetTreeAgeMultiplier(Level level, int2 targetTile)
{
TileData data = level.GetData(targetTile);
if (data == null)
{
return 1f;
}
TreeData customData = data.GetCustomData<TreeData>();
if (customData == null)
{
return 1f;
}
float t = (float)customData.GrowthDays / 8f;
return Mathf.Lerp(5f, 1f, t);
}
}
```
//Save this code as a `.txt` file to keep it for future reference. Each class section is clearly separated for easier navigation.
//LocalPlayer
using System;
using System.Collections;
using System.Collections.Generic;
using System.Runtime.CompilerServices;
using System.Threading;
using Cysharp.Threading.Tasks;
using GameLogic;
using Unity.Mathematics;
using UnityEngine;
using UnityEngine.InputSystem;
using UnityEngine.Localization;
// Token: 0x0200047B RID: 1147
public partial class LocalPlayerController : PlayerController, IUnlockReceiver, IControlMode
{
private GameObject consoleUI;
// Token: 0x0600457A RID: 17786
private new void Start()
{
GameObject localPlayer = this.FindLocalPlayer();
if (localPlayer != null)
{
Transform transform = localPlayer.transform.Find("GameUI/Console/Panel");
this.consoleUI = transform != null ? transform.gameObject : null;
}
if (this.consoleUI == null)
{
Debug.LogError("ConsoleUI GameObject not found. Ensure the hierarchy and paths are correct.");
}
else
{
Debug.Log("ConsoleUI successfully found.");
}
if (this.Input != null)
{
// Original Toggle Button
this.Input.actions["UI/ToggleConsole"].performed += delegate (InputAction.CallbackContext ctx)
{
this.ToggleConsoleUI();
};
// Additional Hardcoded F12 Toggle Button
InputSystem.onEvent += (InputEventPtr eventPtr, InputDevice device) =>
{
if (Keyboard.current != null && Keyboard.current.f12Key.wasPressedThisFrame)
{
this.ToggleConsoleUI();
}
};
}
}
private GameObject FindLocalPlayer()
{
foreach (GameObject obj in GameObject.FindObjectsOfType<GameObject>())
{
if (obj.GetComponent<PlayerController>() != null && obj.name.StartsWith("LocalPlayer"))
{
Debug.Log($"LocalPlayer found: {obj.name}");
return obj;
}
}
Debug.LogError("LocalPlayer not found in the scene.");
return null;
}
private void ToggleConsoleUI()
{
if (consoleUI != null)
{
consoleUI.SetActive(!consoleUI.activeSelf);
Debug.Log($"ConsoleUI is now {(consoleUI.activeSelf ? "visible" : "hidden")}.");
}
else
{
Debug.LogError("ConsoleUI reference is null. Cannot toggle visibility.");
}
}
}
// ConsoleUI Class
// This is the modified code for the ConsoleUI class to allow it to run without restrictions in non-editor or non-debug builds.
using System;
using System.Collections;
using System.Collections.Generic;
using System.Runtime.CompilerServices;
using TMPro;
using UnityEngine;
using UnityEngine.Events;
public partial class ConsoleUI : UIGameObjectWindow, IGameUIListener, IGameUIGetter
{
protected override void Awake()
{
ConsoleUI.IsIgnoringCosts = false;
base.Awake();
// Removed restriction for non-editor or non-debug builds
// if (!Application.isEditor && !Debug.isDebugBuild)
// {
// base.enabled = false;
// return;
// }
this.m_commands.Add("help", new Action<string[]>(this.Help));
this.m_commands.Add("explore", new Action<string[]>(this.Explore));
this.m_commands.Add("freestuff", new Action<string[]>(this.FreeStuff));
this.m_commands.Add("alchemy", new Action<string[]>(this.Alchemy));
this.m_commands.Add("begin", new Action<string[]>(this.Begin));
this.m_commands.Add("tools", new Action<string[]>(this.Tools));
this.m_commands.Add("coppertools", new Action<string[]>(this.CopperTools));
this.m_commands.Add("irontools", new Action<string[]>(this.IronTools));
this.m_commands.Add("moonstonetools", new Action<string[]>(this.MoonstoneTools));
this.m_commands.Add("volcanictools", new Action<string[]>(this.VolcanicTools));
this.m_commands.Add("giveitem", new Action<string[]>(this.GiveItem));
this.m_commands.Add("gi", new Action<string[]>(this.GiveItem));
this.m_commands.Add("ti", new Action<string[]>(this.TakeItem));
this.m_commands.Add("giveallitems", new Action<string[]>(this.GiveAllItems));
this.m_commands.Add("givemoney", new Action<string[]>(this.GiveMoney));
this.m_commands.Add("gm", new Action<string[]>(this.GiveMoney));
this.m_commands.Add("endday", new Action<string[]>(this.EndDay));
this.m_commands.Add("unlockblueprint", new Action<string[]>(this.UnlockBlueprint));
this.m_commands.Add("unlockrecipe", new Action<string[]>(this.UnlockRecipe));
this.m_commands.Add("unlockall", new Action<string[]>(this.UnlockAll));
this.m_commands.Add("unlockalldrawings", new Action<string[]>(this.UnlockAllDrawings));
this.m_commands.Add("unlockallrecipes", new Action<string[]>(this.UnlockAllRecipes));
this.m_commands.Add("unlockallshops", new Action<string[]>(this.UnlockAllShopItems));
this.m_commands.Add("lockrecipe", new Action<string[]>(this.LockRecipe));
this.m_commands.Add("freeblueprints", new Action<string[]>(this.FreeBlueprints));
this.m_commands.Add("settime", new Action<string[]>(this.SetTime));
this.m_commands.Add("givetokens", new Action<string[]>(this.GiveTokens));
this.m_commands.Add("startminigame", new Action<string[]>(this.StartMinigame));
this.m_commands.Add("growforest", new Action<string[]>(this.GrowForest));
this.m_commands.Add("growgrass", new Action<string[]>(this.GrowGrass));
this.m_commands.Add("cleargrass", new Action<string[]>(this.ClearGrass));
this.m_commands.Add("flipinstances", new Action<string[]>(this.FlipInstances));
this.m_commands.Add("getlightamount", new Action<string[]>(this.GetLightAmount));
this.m_commands.Add("rebakenavmesh", new Action<string[]>(this.RebakeNavmesh));
this.m_commands.Add("listunlockedrecipes", new Action<string[]>(this.ListUnlockedRecipes));
this.m_commands.Add("clear", new Action<string[]>(this.ClearLog));
this.m_commands.Add("setrtpc", new Action<string[]>(this.SetRTPC));
this.m_commands.Add("setglobalstate", new Action<string[]>(this.SetGlobalState));
this.m_commands.Add("getglobalstate", new Action<string[]>(this.GetGlobalState));
this.m_commands.Add("setplayerstate", new Action<string[]>(this.SetPlayerState));
this.m_commands.Add("getplayerstate", new Action<string[]>(this.GetPlayerState));
this.m_commands.Add("xpbomb", new Action<string[]>(this.XPBomb));
this.m_commands.Add("dropxp", new Action<string[]>(this.DropXP));
this.m_commands.Add("givexp", new Action<string[]>(this.GiveXP));
this.m_commands.Add("resetcaves", new Action<string[]>(this.ResetCaves));
this.m_commands.Add("resetcave", new Action<string[]>(this.ResetCave));
this.m_commands.Add("startdanger", new Action<string[]>(this.StartDanger));
this.m_commands.Add("setsimulationspeed", new Action<string[]>(this.SetSimulationSpeed));
this.m_commands.Add("setgamespeed", new Action<string[]>(this.SetGameSpeed));
this.m_commands.Add("freeseeds", new Action<string[]>(this.FreeSeeds));
this.m_commands.Add("growcrops", new Action<string[]>(this.GrowCrops));
this.m_commands.Add("spawnasloot", new Action<string[]>(this.SpawnAsLoot));
this.m_commands.Add("moneyasloot", new Action<string[]>(this.MoneyAsLoot));
this.m_commands.Add("teleport", new Action<string[]>(this.Teleport));
this.m_commands.Add("startcutscene", new Action<string[]>(this.StartCutscene));
this.m_commands.Add("godmode", new Action<string[]>(this.EnableGodMode));
this.m_commands.Add("disablegodmode", new Action<string[]>(this.DisableGodMode));
this.m_commands.Add("addtally", new Action<string[]>(this.AddTally));
this.m_commands.Add("fillinventory", new Action<string[]>(this.FillPlayerInventory));
this.m_commands.Add("clearinventory", new Action<string[]>(this.ClearPlayerInventory));
this.m_commands.Add("startquest", new Action<string[]>(this.StartQuest));
this.m_commands.Add("startquestlocal", new Action<string[]>(this.StartQuestLocal));
this.m_commands.Add("startallquestslocal", new Action<string[]>(this.StartAllQuestsLocal));
this.m_commands.Add("forcecompletetask", new Action<string[]>(this.ForceCompleteTask));
this.m_commands.Add("forcecompletetasklocal", new Action<string[]>(this.ForceCompleteTaskLocal));
this.m_commands.Add("forceskiptoendofquest", new Action<string[]>(this.ForceSkipToEndOfQuest));
this.m_commands.Add("forcecompletetrackedtask", new Action<string[]>(this.ForceCompleteTrackedTask));
this.m_commands.Add("forceallcompletetasklocal", new Action<string[]>(this.ForceAllCompleteTaskLocal));
this.m_commands.Add("givealllumas", new Action<string[]>(this.GiveAllLumas));
this.m_commands.Add("upgradetool", new Action<string[]>(this.UpgradeTool));
this.m_commands.Add("ascendtool", new Action<string[]>(this.AscendTool));
this.m_commands.Add("setday", new Action<string[]>(this.SetDay));
this.m_commands.Add("hide", new Action<string[]>(this.HideUI));
this.m_commands.Add("show", new Action<string[]>(this.ShowUI));
this.m_commands.Add("hidegameui", new Action<string[]>(this.HideGameUI));
this.m_commands.Add("showgameui", new Action<string[]>(this.ShowGameUI));
this.m_commands.Add("hideworldui", new Action<string[]>(this.HideWorldUI));
this.m_commands.Add("showworldui", new Action<string[]>(this.ShowWorldUI));
this.m_commands.Add("showwindow", new Action<string[]>(this.ShowWindow));
this.m_commands.Add("hidewindow", new Action<string[]>(this.HideWindow));
this.m_commands.Add("coords", new Action<string[]>(this.PrintCoords));
this.m_commands.Add("next", new Action<string[]>(this.NextTempleRoom));
this.m_commands.Add("ignorecost", new Action<string[]>(this.IgnoreCost));
this.m_commands.Add("spider", new Action<string[]>(this.SpawnSpiderInCave));
this.m_commands.Add("setprof", new Action<string[]>(this.SetProfession));
this.m_commands.Add("allprofs", new Action<string[]>(this.AllProfessions));
this.m_commands.Add("chestcheat", new Action<string[]>(this.ChestCheat));
this.m_commands.Add("resetchests", new Action<string[]>(this.ResetChests));
this.m_commands.Add("setcraftingspeed", new Action<string[]>(this.SetCraftingSpeed));
this.m_commands.Add("setcropspeed", new Action<string[]>(this.SetCropSpeed));
this.m_commands.Add("spawnspider", new Action<string[]>(this.SpawnSpider));
this.m_commands.Add("spawnbee", new Action<string[]>(this.SpawnBee));
this.m_commands.Add("killspiders", new Action<string[]>(this.KillSpiders));
this.m_commands.Add("killbear", new Action<string[]>(this.KillBear));
this.m_commands.Add("spawnghost", new Action<string[]>(this.SpawnGhost));
this.m_commands.Add("spawnblueprint", new Action<string[]>(this.SpawnBlueprint));
this.m_commands.Add("unstuck", new Action<string[]>(this.Unstuck));
this.m_commands.Add("teleporttotile", new Action<string[]>(this.TeleportPlayerToTile));
this.m_commands.Add("bait", new Action<string[]>(this.AddBait));
this.m_commands.Add("printwindowstate", new Action<string[]>(this.PrintWindowState));
this.m_commands.Add("enablewindowlog", new Action<string[]>(this.EnableWindowLog));
this.m_commands.Add("disablewindowlog", new Action<string[]>(this.DisableWindowLog));
this.m_commands.Add("nuke", new Action<string[]>(this.Nuke));
this.m_commands.Add("loadlevel", new Action<string[]>(this.AdditiveLoadLevel));
this.m_input.onValueChanged.AddListener(new UnityAction<string>(this.UpdateSuggestions));
TMP_InputField input = this.m_input;
input.onValidateInput = (TMP_InputField.OnValidateInput)Delegate.Combine(input.onValidateInput, new TMP_InputField.OnValidateInput(this.IgnoreBackquote));
}
}